How To: Create a new Outlook Personal Folders store and add it to the current profile
Sometimes developers need to add an additional store in Outlook for archiving e-mails, making backup copies of email conversations etc. The AddStore method of the Namespace class of the Outlook Object Model provides the required functionality. It adds a personal folders file (.pst) to the current profile. So, let’s look at this method in depth! I’ll provide code samples both for VSTO developers and those who develop with Add-in Express for Office and .net.
A personal folders store. What is it?
Typically a personal folders store represents a root folder in the Outlook Navigation pane. After you run a sample code which adds stores you can see a similar picture in your Outlook profile. The screenshots were captured on my PC with Windows 7 x64 and Outlook 2010 x64.
Also you may find the results of our tests in the Mail section of the Control Panel applet.
- 1. Go to User Accounts and click Mail.
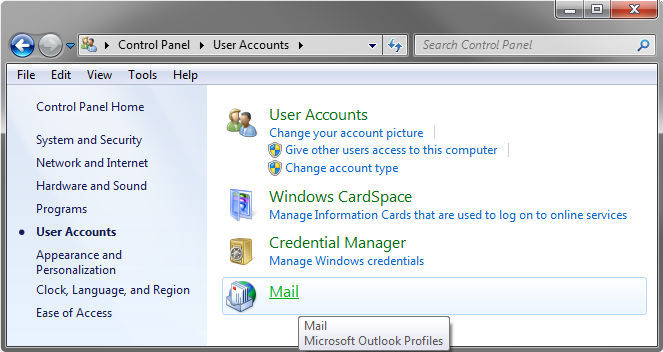
-
2. Choose an appropriate account.
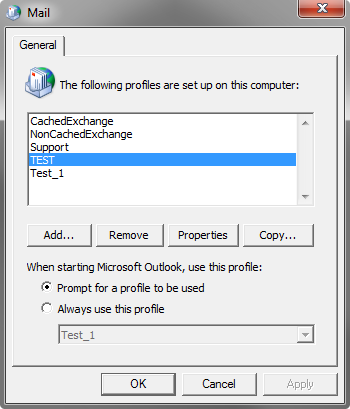
- 3. Press the Data Files… button to see all available data files.
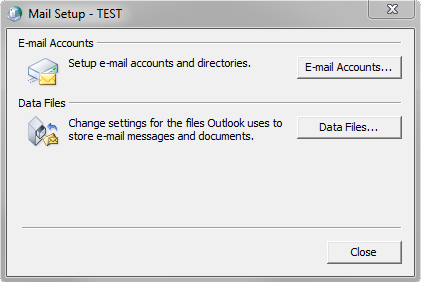
- 4. Here you are! Now you can see two additional stores that have been added using the sample code.
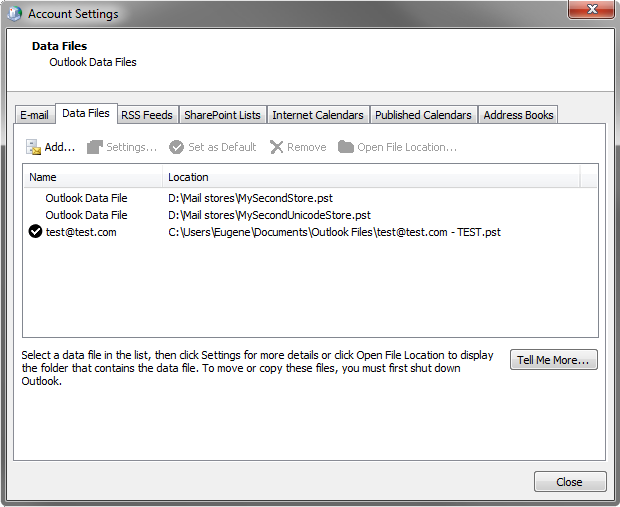
Coding… coding… coding…
The AddStore method accepts a string which represents a file path to the Outlook .pst file. If there is no such .pst file on the hard drive it will be created automatically. I would like to point out the fact that the AddStore method doesn’t return a store object. It doesn’t return anything at all; the method just does its job. That is because the Stores collection and the Store class were introduced in Outlook 2007. Outlook 2010 brought some new methods and properties to the Store class like Categories, IsConversationEnabled and GetDefaultFolder, etc.
Outlook 2003 introduced the AddStoreEx method which requires a second parameter. It can be one of the following constants of the OlStoreType enumeration: olStoreDefault, olStoreUnicode and olStoreANSI.
- olStoreDefault – allows you to create a .pst file in the default format which is compatible with the mailbox mode (the cached or non-cached exchange mode).
- olStoreUnicode – allows you to add a new .pst file to the user’s profile that has a greater capacity (than the ANSI format provides) and supports Unicode data. The Unicode format of the .pst file was introduced with Outlook 2003.
- olStoreANSI – allows you to add a new .pst file which is compatible with all versions of Outlook including Outlook 2000 and 2002, but it doesn’t fully support Unicode data.
You just need to pass an instance of the Outlook Application class to the AddNewPersonalStore method to get it running.
C# & Add-in Express:
private void AddNewPersonalStore(Outlook._Application OutlookApp) { Outlook.NameSpace ns = null; try { ns = OutlookApp.GetNamespace("MAPI"); ns.AddStore(@"D:\Mail stores\MySecondStore.pst"); // ns.AddStoreEx(@"D:\Mail stores\MySecondUnicodeStore.pst", // Outlook.OlStoreType.olStoreUnicode); } catch (Exception ex) { MessageBox.Show("Error: " + ex.Message); } finally { if (ns != null) Marshal.ReleaseComObject(ns); } }
C# & VSTO:
using System.Runtime.InteropServices; using System.Windows.Forms; // private void AddNewPersonalStore(Outlook.Application Application) { Outlook.NameSpace ns = null; try { ns = Application.GetNamespace("MAPI"); ns.AddStore(@"D:\Mail stores\MySecondStore.pst"); // ns.AddStoreEx(@"D:\Mail stores\MySecondUnicodeStore.pst", // Outlook.OlStoreType.olStoreUnicode); } catch (Exception ex) { MessageBox.Show("Error: " + ex.Message); } finally { if (ns != null) Marshal.ReleaseComObject(ns); } }
VB.NET & Add-in Express:
Private Sub AddNewPersonalStore(ByRef OutlookApp As Outlook._Application) Dim ns As Outlook.NameSpace = Nothing Try ns = OutlookApp.GetNamespace("MAPI") ns.AddStore("D:\Mail stores\MySecondStore.pst") ' ns.AddStoreEx("D:\Mail stores\MySecondUnicodeStore.pst", ' Outlook.OlStoreType.olStoreUnicode) Catch ex As Exception MsgBox("Error: " + ex.Message) Finally If Not IsNothing(ns) Then Marshal.ReleaseComObject(ns) End Try End Sub
VB.NET & VSTO:
Imports System.Runtime.InteropServices ' Private Sub AddNewPersonalStore(ByRef Application As Outlook.Application) Dim ns As Outlook.NameSpace = Nothing Try ns = Application.GetNamespace("MAPI") ns.AddStore("D:\Mail stores\MySecondStore.pst") ' ns.AddStoreEx("D:\Mail stores\MySecondUnicodeStore.pst", ' Outlook.OlStoreType.olStoreUnicode) Catch ex As Exception MsgBox("Error: " + ex.Message) Finally If Not IsNothing(ns) Then Marshal.ReleaseComObject(ns) End Try End Sub
If you run the code in Outlook 2010, you may notice that the following calls are identical:
AddStore(@"D:\Mail stores\MySecondStore.pst"); ... AddStoreEx(@"D:\Mail stores\MySecondUnicodeStore.pst", Outlook.OlStoreType.olStoreUnicode);
Also, I developed a simple windows forms application which adds a new store to the Outlook profile. Here is the code. Please don’t forget to add a COM reference to Outlook if you want to get the code running.
C# & Windows Forms Application:
using Outlook = Microsoft.Office.Interop.Outlook; using System.Runtime.InteropServices; // private void AddNewStoreToOutlook(string pathToPst) { Outlook.Application app = null; Outlook.NameSpace ns = null; try { app = new Outlook.Application(); ns = app.GetNamespace("MAPI"); ns.Logon(); ns.AddStore(pathToPst); ns.Logoff(); app.Quit(); } catch (Exception ex) { MessageBox.Show("Error: " + ex.Message); } finally { if (ns != null) Marshal.ReleaseComObject(ns); if (app != null) Marshal.ReleaseComObject(app); } }
VB.NET & Windows Forms Application:
Imports System.Runtime.InteropServices Imports Outlook = Microsoft.Office.Interop.Outlook ' Private Sub AddNewStoreToOutlook(pathToPst As String) Dim app As Outlook.Application = Nothing Dim ns As Outlook.NameSpace = Nothing Try app = New Outlook.Application() ns = app.GetNamespace("MAPI") ns.Logon() ns.AddStore(pathToPst) ns.Logoff() app.Quit() Catch ex As Exception MessageBox.Show("Error: " + ex.Message) Finally If Not IsNothing(ns) Then Marshal.ReleaseComObject(ns) If Not IsNothing(app) Then Marshal.ReleaseComObject(app) End Try End Sub
As you may see, the Logon and Logoff methods were used in case of a standalone application. That is because add-ins do their job after a user has logged into an Outlook profile.
See you on our forums and in the e-mail support!
18 Comments
HI Astafiev:
I have some problem about MAPIStore.I want to add a new store,and set it as default ,but I donot know how to set it as default after I created a new pst file by ‘app.Session.AddStoreEx?xxxx,xxxx?‘.
Could you help me and tell me how can i to resolve.
Thank you very much.
Hi Maxinm,
The Outlook Object Model doesn’t provide any property or method for setting the default store. However, the read-only DefaultStore property of the Namespace class can be used to get the default store in the profile.
Hi Eugene,
I would like to create a vbs to automatically copy OST file to PST file in Outlook 2010 (both 32-bit and 64-bit.) I got below code, but it’s not working, could you please take a look why it’s not working. Thank you in advance.
‘Define some constants’
Const BACKUP_PATH = “C:\temp\Backups\”
‘Create some variables
Dim strBackupFileName, objFSO, olkApp, olkSes, olkRootFolder, olkFolder, olkFolderCopy, olkBackup
strBackupFileName = “LS ” & Year(Date) & “-” & Month(Date) & “-” & Day(Date) & “.pst”
‘Connect to Outlook’
Set olkApp = CreateObject(“Outlook.Application”)
Set olkSes = olkApp.GetNamespace(“MAPI”)
olkSes.Logon olkApp.DefaultProfileName
‘Main routine’
On Error Resume Next
olkSes.AddStore BACKUP_PATH & strBackupFileName
Set olkBackup = olkSes.Stores.Item(“Personal Folders”)
olkBackup.Name = strBackupFileName
olkSes.RemoveStore olkBackup
olkSes.AddStore BACKUP_PATH & strBackupFileName
Set olkBackup = olkSes.Stores.Item(strBackupFileName)
Set olkRootFolder = olkSes.DefaultStore.GetRootFolder
For Each olkFolder In olkRootFolder.Folders
Set olkFolderCopy = olkFolder.CopyTo(olkBackup)
Next
olkSes.RemoveStore olkBackup
On Error Goto 0
‘Disconnect from Outlook’
olkSes.Logoff
‘Clean-up’
Set objFSO = Nothing
Set olkBackup = Nothing
Set olkRootFolder = Nothing
Set olkFolder = Nothing
Set olkFolderCopy = Nothing
Set olkSes = Nothing
Set olkApp = nothing
Hi Larry,
Do you get any errors or exceptions?
Anyway, I can’t give the exact answer off the top of my head, it requires debugging and researching.
Please drop me an e-mail to our support address and I will continue to help you with this issue. You can find the support e-mail address in the readme.txt file (it is included in our product packages).
Hi Eugene,
Thank you for the response.
The code works till Set olkBackup = olkSes.Stores.Item(strBackupFileName)
From Set olkBackup = olkSes.Stores.Item(strBackupFileName), it stopped working.
I will drop you an email.
Hi Larry,
Do you get any errors or exceptions?
Of course, please contact me via the support e-mail address and we will continue our investigation.
Hello friends,
I want to add folder in personal folder ex.
———————————————-
Personal Folder
|
Folder1
|
Folder2
|
etc…
———————————————
I can add first time but at second time it gives exception as “can’t create folder”
My code is…
———————————————
Dim objOL As New Outlook.Application
Dim myFolder As Outlook.MAPIFolder = objOL.Session.Folders.Item(1).Folders.Add(“Folder1”)
——————————————————-
please help me…
Hi Naresh,
Please take a look at the How To: Set a custom icon for an Outlook folder in the Navigation Pane article on our technical blog for more information on this. Pay special attention to the SetUpFolder sub (creates a folder if it doesn’t exist).
can you help? i would like to create a button in Visual Basic Studio Express that would create a personal folder in outlook, automating the process for users, instead of the users going to file/data file Management and so forth in outlook to create the personal folder.
I suggest that you use the sample project “Your First Microsoft Office COM Add-in”. You can find it in the archive labeled “Add-in Express for Office and .NET sample projects” at https://www.add-in-express.com/downloads/adxnet.php. You’ll need to call the AddNewPersonalStore() method (see above) in the Click event of that button.
Hi, i download the zip file that contains “Your first Microsoft Office com Add-in” which module would i am to run to get the script to create the button? AddinModule? can you please?
thank you
Hmm, this sample provides a complete source code of the add-in which is discussed in the Add-in Express documentation. If you are a programmer and if you have Add-in Express, you are able to modify the source code as I suggested earlier, to build an add-in and to deploy it.
How to define a new data file outlook as default by vb.net?
Hi Sanderson,
I am afraid it is impossible because the DefaultStore property is read-only.
Hi
When we create .pst file through the object model we find it is then impossible to se the .WebFolderUrl property (we can assign a value but it doesnt get updated on the store object. Has anyone go a workaround for this?
Hello Adrian,
Please see https://support.microsoft.com/kb/923933. Note that 12 in that registry key is version-dependent: 12 – 2007, 14 – 2010, 15 – 2013. Hope this helps.
Thanks, yes I have stumbled across this too and setting the key certainly unlocks the desired functionality. So then the question is it OK to add / update this registry key on install (or are we opening some great security risk)and if it is OK do we devise our own way of doing the key in our installer (or is there anything built into add in express that will do it for us)?
Add-in Express doesn’t have any built-in means to create this key. Microsoft doesn’t suggest the way to choose, so you are free. Actually, I think some administrators may prefer you not to use that setting, so you may need to contact your administrator before installing the add-in.